
The Swift Cookbook of Code
Get the Swift Cookbook
This course is a compilation of hundreds of Swift code solutions neatly organized in sections for fast reference when you need it.
Each lecture will highlight example code in a brief video, and demonstrate how to use it correctly, as well as best practices for its implementation.
Anyone who writes Swift code needs this library in their coding toolbox for fast reference and usage.
Please note: This Swift Cookbook course will not teach you the Swift language, or how to code,
You should already have completed Paul Hudson's Swift tutorials at HackingWithSwift.com, or the Swift Mastery Course, or have written your own existing Swift apps.
Navigate through hundreds of code examples very quickly to get the code solution you need.
What is the requirement?
You should already be familiar with Swift and the app building process.
Who should take this course?
This course was designed with the developer in mind, as well as the student who is learning and has a good grasp of Swift and Cocoa Touch.
About the Instructor
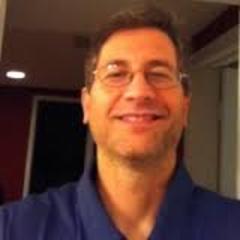
Hi my name is Stephen DeStefano, I am an Apple developer and have been creating apps for 5 years now, I have numerous apps in the app store, and i continue to stay current and grow with Apple as technology continues to evolve. My goal here is to create video instruction that makes it easy for students to work with Apple's new language called swift, and incorporate that knowledge into creating some pretty amazing apps for the apps store. If your thinking that its too difficult, then come code along with me and see how easy it is to pick this language up and turn your ideas into code, then into working apps.
Entrepreneur
Have successfully exited his startup in 2011. After joining Rakuten and Recruit helping in their corporate venture capital division. Masa have realised the critical issue of lack of vital education in Japan market. Which lead to go back to the start up scene building an ed-tech company BeSomebody.
BeSomeoboy focus on creating vital education online such as web programming, IOS programming and Excel.
Course content
Section 1:Intoroduction | ||||
1 | Introduction - How to use this course | Preview | 2:13 | |
Section 2:Arryays | ||||
2 | How create multi dimensional arrays? | 1:15 | ||
3 | Count objects in a set(NSCountedSet) | 1:05 | ||
4 | Enumerate items in an array | 0:22 | ||
5 | Find an item in an array using indexOf | 0:15 | ||
6 | Join array of strings | 0:24 | ||
7 | Loop through an array in reverse | 0:51 | ||
8 | Loop through items in array | 0:27 | ||
9 | Shuffle an array in iOS 8 and below | 0:26 | ||
10 | Shuffle an array in iOS 9(GameplayKit) | 0:30 | ||
11 | Sort an array using sort | 0:38 | ||
12 | Tell if an array contains an object | 0:22 | ||
Section 3:CALayer | ||||
13 | Add a border outline color to a UIView | 0:30 | ||
14 | Create keyframe animations | 1:32 | ||
15 | Draw color gradients (CAGradientLayer) | 1:24 | ||
16 | Draw shapes using CAShapeLayer | 0:36 | ||
17 | Emit particles using CAEmitterLayer | 1:08 | ||
18 | How to round the corners of a UIView | 0:42 | ||
Section 4:Core Graphics | ||||
19 | Calculate the distance between 2CGpoints | 0:45 | ||
20 | Calculate the Manhattan distance | 0:32 | ||
21 | Compare(two CGRects,CGRectEqualToRect) | 0:22 | ||
22 | Draw a circle using Core Graphics | 0:36 | ||
23 | Draw a square using Core Graphics | 0:47 | ||
24 | Draw a text string using Core Graphics | 0:26 | ||
25 | How to draw lines in Core Graphics | 0:59 | ||
26 | Find a scale(CGAffineTransform) | 0:12 | ||
27 | Find the rotation(CGAffineTransform) | 0:12 | ||
28 | Find the translation(CGAffineTransform) | 0:13 | ||
29 | Render a PDF to an image | 0:44 | ||
30 | Draw a UIImage differently | 1:43 | ||
Section 5:Games | ||||
31 | Add physics to an SKSpriteNode | Preview | 0:46 | |
32 | Add pixel perfect physics | 0:27 | ||
33 | Color an SKSpriteNode(colorBlendFactor) | 0:34 | ||
34 | Create 3D audio sound using SKAudioNode | 1:15 | ||
35 | Create shapes using SKShapeNode | 0:28 | ||
36 | Emit particles using SKEmitterNode | 0:58 | ||
37 | Generate a random number(GKRandomSource) | 1:04 | ||
38 | Roll a dice | 0:37 | ||
39 | Run SKActions in a group | 0:27 | ||
40 | Run SKActions in a sequence | 0:25 | ||
41 | Stop SKPhysicsBody responding to physics | 0:43 | ||
42 | Write text using SKLabelNode | 0:43 | ||
43 | Find a touches location in a node | 0:38 | ||
44 | Change SKScene with a transition | 0:29 | ||
Section 6:Language | ||||
45 | "Ambiguous reference to member ... " | 1:05 | ||
46 | "ViewController has no initializers" | 1:18 | ||
47 | Check for valid method input | 1:06 | ||
48 | Check the Swift version at compile time | 0:37 | ||
49 | Compare two tuples for the equality | 0:30 | ||
50 | Convert a float to a CGFloat | 0:30 | ||
51 | Convert a float to an int | 0:17 | ||
52 | Convert a string to a double | 0:12 | ||
53 | Convert a string to a float | 0:12 | ||
54 | Convert a string to an int | 0:18 | ||
55 | Convert a string to an NSString | 0:44 | ||
56 | Convert an int to a float | 0:17 | ||
57 | Convert an int to a string | 0:23 | ||
58 | Create an Objective C bridging header | 1:02 | ||
59 | Delay execution of code(defer keyword) | 0:36 | ||
60 | Find the maximum of three numbers | 0:14 | ||
61 | Find the maximum of two numbers | 0:08 | ||
62 | Find the minimum of three numbers | 0:13 | ||
63 | Find the minimum of two numbers | 0:11 | ||
64 | Force your program to crash with assert | 1:28 | ||
65 | Install a beta version of swift | 3:47 | ||
66 | Unwrap an optional in swift | 0:53 | ||
67 | Use compiler directives | 0:45 | ||
68 | Use the try/catch to handle exceptions | 1:34 | ||
69 | Tips switching to Swift | 2:38 | ||
70 | Using stride | 1:08 | ||
71 | What are lazy variables | 3:21 | ||
72 | What are property observers | 0:21 | ||
73 | What is a CGFloat ? | 0:26 | ||
74 | What is a protocol ? | 1:14 | ||
75 | What is the nil coalescing operator ? | 1:00 | ||
76 | What is trailing closure syntax ? | 1:14 | ||
Section 7:Libraries | ||||
77 | Get a Cover Flow effect on iOS | 1:48 | ||
78 | Make look more attractive | 3:02 | ||
79 | Parse JSON using SwiftyJSON | 2:55 | ||
Section 8:Location | ||||
80 | Make an iPhone transmit an iBeacon | 1:21 | ||
81 | Button to an MKMapView annotation | 1:10 | ||
82 | Add an MKMapView using MapKit | 0:42 | ||
83 | Detect iBeacons | 4:38 | ||
84 | Find directions | 1:20 | ||
85 | Request a users location only ones | 0:25 | ||
Section 9:Media | ||||
86 | Detect faces in a UIImage | Preview | 0:28 | |
87 | Choose a photo from the camera roll | 0:49 | ||
88 | Convert text to speech | 0:41 | ||
89 | Create a barcode | 0:25 | ||
90 | Create a PDF417 barcode | 0:27 | ||
91 | Create a QR code | 0:29 | ||
92 | Filter images(Core Image and CIFilter) | 0:35 | ||
93 | Highlight text to speech words be read | 1:06 | ||
94 | Make resizable images | 0:48 | ||
95 | Play sounds using AVAudioPlayer | 1:31 | ||
96 | Record audio using AVAudioRecorder | 3:13 | ||
97 | Record user videos using ReplayKit | 1:12 | ||
98 | Render a UIView to a UIImage | 0:21 | ||
99 | Save a UIImage to a file | 1:06 | ||
100 | Scan a barcode | 0:54 | ||
101 | Scan a QR code | 1:08 | ||
102 | Make a torch(on the camera flashlight) | 0:46 | ||
103 | Write to the iOS photo album | 0:58 | ||
Section 10:Strings | ||||
104 | Capitalize words in a string | 0:15 | ||
105 | Convert a string to lowercase letters | 0:08 | ||
106 | Convert a string to uppercase letters | 0:09 | ||
107 | Detect a URL in a String(NSDataDetector) | 0:38 | ||
108 | Get the length of a string | 0:06 | ||
109 | Load a string from a file in your bundle | 0:32 | ||
110 | Load a string from a website URL | 0:50 | ||
111 | Loop through letters in a string | 0:21 | ||
112 | Measure a string | 0:15 | ||
113 | Parse a sentence(NSLinguisticTagger) | 0:56 | ||
114 | Repeat a string | 0:22 | ||
115 | Reverse a string using reverse() | 0:12 | ||
116 | Specify floating-point precision | 0:52 | ||
117 | Split a string into an array | 0:20 | ||
118 | Test localization | 0:54 | ||
119 | Trim whitespace in a string | 0:31 | ||
120 | Combine strings, integers and doubles | 0:28 | ||
121 | Match regular expressions in strings | 1:09 | ||
122 | Replacing text in a string | 0:26 | ||
123 | Save a string to a file on the disk | 0:50 | ||
Section 11:System | ||||
124 | Read from the command line | 1:08 | ||
125 | Cache data using NSCache | 0:50 | ||
126 | Cancel a delayed performSelector call | 1:31 | ||
127 | Copy objects in Swift using copy | 1:22 | ||
128 | Copy text to the clipboard(UIPasteboard) | 0:26 | ||
129 | Create a peer to peer network | 2:41 | ||
130 | Create rich formatted text strings | 0:41 | ||
131 | Detect low power mode is enabled | 0:44 | ||
132 | Detect when app moves to the background | 0:46 | ||
133 | Detect which country a user is in | 1:25 | ||
134 | Find the users documents directory | 0:30 | ||
135 | Format dates with an ordinal suffix | 0:23 | ||
136 | Generate a random identifier | 0:30 | ||
137 | Generate random numbers in iOS 8 | 0:31 | ||
138 | Handle the HTTPS requirements in iOS 9 | 2:26 | ||
139 | Identify an iOS device uniquely | 0:49 | ||
140 | Insert images into an attributed string | 1:22 | ||
141 | Make an action repeat using NSTimer | 0:53 | ||
142 | Make tappable links(NSattributedString) | 0:55 | ||
143 | Open a URL in Safari | 0:28 | ||
144 | Parse JSON using NSJSONSerialization | 1:15 | ||
145 | Pass data between two view controllers | 1:25 | ||
146 | Post messages using NSNotificationCenter | 1:09 | ||
147 | Read the contents of a directory | 0:29 | ||
148 | Run code after a delay | 0:55 | ||
149 | Run code asynchronously | 0:52 | ||
150 | Run code at a specific time | 1:10 | ||
151 | Run code on the main thread | 0:25 | ||
152 | Save and load objects | 0:50 | ||
153 | Save user settings(NSUserDefaults) | 1:42 | ||
154 | Set local alerts(UILocalNotification) | 2:04 | ||
155 | Spell out numbers | 0:36 | ||
156 | Stop the screen from going to sleep | 0:22 | ||
157 | Store NSUserDefaults options in iCloud | 2:07 | ||
158 | Synchronize code to drawing | 1:18 | ||
159 | Read accelerometer data | 1:01 | ||
160 | Index content in your app | 1:43 | ||
161 | Authenticate users by fingerprint | 1:35 | ||
162 | Add letterpress effect to text | 0:24 | ||
Section 12:UIColor | ||||
163 | Convert a hex color to a UIColor | 0:53 | ||
164 | Convert a HTML name string | 1:35 | ||
165 | Create custom colors | 1:06 | ||
Section 13:UlKit | ||||
166 | UITabBarController tabs can be edited | Preview | 1:13 | |
167 | "Failed to obtain from its DataSource" | 1:29 | ||
168 | "Unable to dequeue with identifier" | 2:01 | ||
169 | Show a modal view controller | Preview | 1:55 | |
170 | Add a bar button to a navigation bar | Preview | 1:08 | |
171 | Add a button to a UITableViewCell | 0:19 | ||
172 | Add a custom view to a UIBarButtonItem | Preview | 0:26 | |
173 | Add a section header to a table view | 0:20 | ||
174 | Add a shadow to a UIView | Preview | 1:03 | |
175 | Add a UITextField to a UIAlertController | 0:24 | ||
176 | Add blur and vibrancy | Preview | 1:21 | |
177 | Add flexible space to a UIBarButtonItem | 0:40 | ||
178 | Add iAd to a view controller | 2:01 | ||
179 | Add retina and retina HD graphics | 1:54 | ||
180 | Add a quick action for 3D Touch | 2:42 | ||
181 | Adjust to fit the keyboard | 0:53 | ||
182 | Adjust image content mode | 1:14 | ||
183 | Animate views(animateWithDuration) | 0:58 | ||
184 | Animate views with spring dampening | 1:02 | ||
185 | Animate when your size class changes | 2:24 | ||
186 | Bring a subView to the front of a UIView | 0:25 | ||
187 | Change the scroll indicator inset | 0:33 | ||
188 | Check if a string is spelled correctly | 0:31 | ||
189 | Convert a CGPoint to another view | 0:35 | ||
190 | Create a page curl affect | 1:58 | ||
191 | Create a parallax effect in UIKit | 0:27 | ||
192 | Create auto layout constraints in code | 1:42 | ||
193 | Create custom menus | 1:08 | ||
194 | Create popover menus | 0:57 | ||
195 | Customize swipe edit buttons | 0:58 | ||
196 | Deselect a UITableViewCell | 0:51 | ||
197 | Detect a double tap gesture | 0:20 | ||
198 | Detect edge swipes | 0:22 | ||
199 | Detect when the back button is tapped | 0:46 | ||
200 | Dim the screen | 0:31 | ||
201 | Draw custom views in interface builder | 0:59 | ||
202 | Find a UIView subview using viewWithTag | 0:47 | ||
203 | Flip a UIView with 3D effect | 0:29 | ||
204 | Give a background image | 0:34 | ||
205 | Give a background color | 0:19 | ||
206 | Give UITableViewCells a selected a color | 0:31 | ||
207 | Hide passwords in a UITextField | 0:19 | ||
208 | Hide the navigation bar | 0:36 | ||
209 | Hide the navigation bar(hidesBarsOnTap) | 0:31 | ||
210 | Hide the status bar | 0:41 | ||
211 | Tap on while editing is enabled | 0:40 | ||
212 | Limit the number of characters | 0:57 | ||
213 | Load a HTML string | 0:41 | ||
214 | Make a button glow when tapped | 0:25 | ||
215 | Make clear button appear in textfield | 0:23 | ||
216 | Make the master pane always visible | 0:25 | ||
217 | Make UITableViewCell go edge to edge | 0:43 | ||
218 | Make UITableViewCells resize to content | 0:49 | ||
219 | Measure touch strengths using 3D Touch | 0:43 | ||
220 | Pad by setting it's text container inset | 0:20 | ||
221 | Print using UIActivityViewController | 1:03 | ||
222 | Put a background picture behind | 0:16 | ||
223 | Read a title from UIPickerView | 1:26 | ||
224 | Recolor UIImages | 0:36 | ||
225 | Register a cell for View reuse | 0:54 | ||
226 | Remove a UIView from its superview | 0:20 | ||
227 | Remove cells from a UITableView | 0:45 | ||
228 | Respond to the device being shaken | 0:26 | ||
229 | Scale, stretch, move and rotate UIViews | 0:47 | ||
230 | Send an email | 0:52 | ||
231 | Set custom title view in UINavigationBar | 0:32 | ||
232 | Set different widths for a elements | 0:44 | ||
233 | Set prompt text in a navigation bar | 0:28 | ||
234 | Set the tabs in a UITabBarController | 0:36 | ||
235 | Set the tint color of a UIView | 0:50 | ||
236 | Share content with ... | 0:43 | ||
237 | Share with UIActivityViewController | 1:09 | ||
238 | Show a toolbar | 0:35 | ||
239 | Stop auto layout and resizing masks | 0:45 | ||
240 | Stop empty row separators | 0:43 | ||
241 | Stop users selecting text | 0:22 | ||
242 | Stop view going under the navigation bar | 0:38 | ||
243 | Style the font in UINavigationBars title | 0:35 | ||
244 | Support pinch to zoom in a UIScrollView | 0:45 | ||
245 | Swipe to delete UITableViewCells | 1:08 | ||
246 | Use Dynamic Type to resize apps text | 0:55 | ||
247 | Adjust values in interface builder | 1:31 | ||
248 | Use light text color in the status bar | 0:44 | ||
249 | Show web pages in your app | 1:20 | ||
250 | Find touches location in a view | 0:37 | ||
251 | Run JavaScript on a UIWebView | 0:31 | ||
252 | What are size classes | 2:07 | ||
253 | Different UIStackView distribution types | 1:29 | ||
254 | What is content compression resistance | 0:57 | ||
255 | Message Simulator user has requested | 0:53 | ||
256 | Case of can't register for notifications | 1:05 | ||
Section 14:WKWebView | ||||
257 | Run javaScript on a WKWebView | 0:21 | ||
258 | Enable back and forward swiping gestures | 0:25 | ||
259 | Monitor WKWebView Page load progress | 1:13 | ||
260 | UIWebView and WKWebView | 1:17 | ||
Section 15:Xcode | ||||
261 | Create exception break points in Xcode | 0:55 | ||
262 | Debug view layouts in Xcode | 0:58 | ||
263 | Load assets from Xcode assets catalogs | 1:08 | ||
Section 16:Hacking With Swift - A must have resource | ||||
264 | Hacking with swift - course wrapup | 3:46 |